How to Sum Arrays in JavaScript (using reduce method)
So you have an array with a bunch of values you want to sum up. To do that, we will use the reduce method.
The reduce()
method reduces an array and returns a single value.
The reduce()
method will iterate through every element in the array. We use two parameters. The first is a function and the second is the initial value.
We set the initialValue
to 0.
In the function, we have two parameters. totalValue
and currentValue
.
totalValue
is the value returned from the previous iteration or the initial value.
currentValue
is the current value in the iteration.
Let’s look at an example to clarify:
let numbers = [1, 2, 3, 4];
let initialValue = 0;
let sum = numbers.reduce((totalValue, currentValue) => {
console.log(`totalValue: ${totalValue}, currentValue: ${currentValue}`)
return totalValue + currentValue
}, initialValue);
console.log("sum", sum);
I’m logging to the console here. That way we can see what is happening for each iteration.
This is the output:
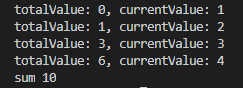
In the first iteration, we see that totalValue
is 0, which is the initial value. The currentValue
is the first element in the array which is 1. We return 0 + 1 which is 1.
The second iteration, we see that totalValue
is 1. That is the value we returned in the previous iteration. currentValue
is 2 which is the second element in the array. We return 1+2 which is 3 and that will be the value of totalValue
in the next iteration.
This continues until we have iterated through the array.
To shorten the code we can refactor the code to look like this:
let numbers = [1, 2, 3, 4];
let initialValue = 0;
let sum = numbers.reduce((totalValue, currentValue) => totalValue + currentValue, initialValue);
How to Sum an Array with Objects
To sum an array with values of an object we have almost the same code.
let initialValue = 0;
let objects = [{ x: 1 }, { x: 2 }, { x: 3 }, { x: 4 }];
let sum = objects.reduce((totalValue, currentValue) => {
console.log(`totalValue: ${totalValue}, currentValue: ${currentValue}`)
return totalValue + currentValue.x;
}, 0);
console.log(sum);
This will return the following output:
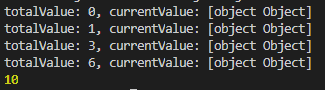
Her we have an array of objects and each has a property x.
totalValue
is the sum accumulator as before.
currentValue
is the current element of the array. Now, this element is an object. So the only thing we have to do different in this example is to add the totalValue
with the x property of the current value.
Final Words
In my opinion, the best way to sum arrays in JavaScript. It is the cleanest way I’ve found even if it is not that intuitive at first. I also like that it is 100% vanilla JavaScript.
There are of course other ways to sum up arrays in JavaScript:
- Loop through the array with a for-loop and append the value
- Use libraries such as Lodash which has a sum function.